Welcome to the ultimate GetX State Management cheat sheet! In this blog post, I will share the GetX State Management cheat sheet that will help you remember the basic basics of GetX state management in Flutter. It will enhance your Flutter development journey.
Table of Contents
Download GetX State Management Cheat Sheet PDF
Download the GetX State Management cheat sheet PDF. It will boost your Flutter skills, covering key concepts for efficient app development. Purchase now and support our efforts!
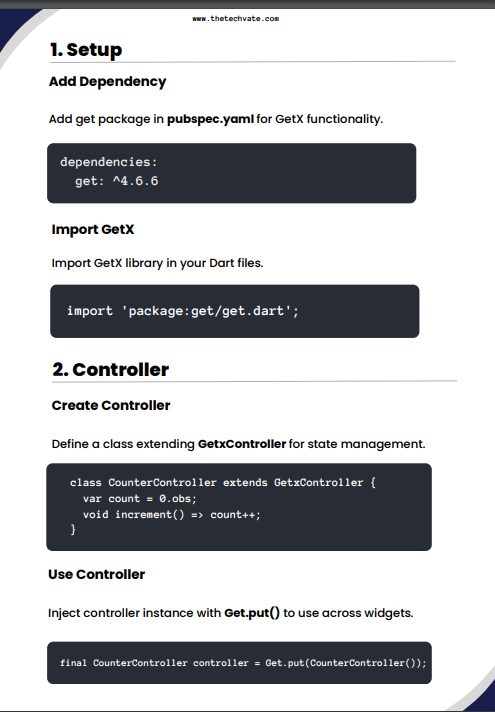
1. Setup
Add Dependency
Add get package in pubspec.yaml for GetX functionality.
dependencies:
get: ^4.6.5
Import GetX
Import GetX library in your Dart files.
import 'package:get/get.dart';
2. Controller
Create Controller
Define a class extending GetxController for state management.
class CounterController extends GetxController {
var count = 0.obs;
void increment() => count++;
}
Use Controller
Inject controller instance with Get.put() to use across widgets.
final CounterController controller = Get.put(CounterController());
3. Observables
Reactive Variables
Use .obs to make a variable observable for state changes.
var count = 0.obs; // or RxInt(0)
var name = ''.obs; // or RxString('')
Update UI with Obx
Use Obx widget to rebuild UI on reactive variable changes.
Obx(() => Text("Count: ${controller.count}"));
4. Routes
Simple Route Navigation
Navigate to new pages using the Get.to() method.
Get.to(SecondPage());
Named Route Navigation
Use Get.toNamed() for navigation with named routes.
Get.toNamed('/second');
Route Setup
Define routes in GetMaterialApp for app-wide navigation.
GetMaterialApp(
initialRoute: '/',
getPages: [
GetPage(name: '/', page: () => HomePage()),
GetPage(name: '/second', page: () => SecondPage()),
],
);
5. Dependency Injection
Inject Dependency
Use Get.lazyPut() to lazy initialize controllers or services.
Get.lazyPut(() => Controller());
Find Dependency
Retrieve controller instances using Get.find().
final controller = Get.find();
6.Dialogs, Snackbars, Bottom Sheets
Show Dialog
Display custom dialogs with Get.defaultDialog().
Get.defaultDialog(title: "Title", content: Text("Content"));
Show Snackbar
Use Get.snackbar() to show snackbars for messages.
Get.snackbar('Title', 'Message');
Show Bottom Sheet
Display bottom sheets using Get.bottomSheet().
Get.bottomSheet(Container(child: Text("Bottom Sheet")));
7. Other Utilities
Show Loading
Display a loading dialog with Get.dialog().
Get.dialog(
Center(
child: CircularProgressIndicator()),
barrierDismissible: false
);
Close Dialogs, Bottom Sheets, etc.
Use Get.back() to close any open widget.
Get.back();
8. Form Validation
Controller with Validators
Create validators inside controllers for form fields.
class LoginController extends GetxController {
var email = ''.obs;
var password = ''.obs;
String? validateEmail(String value) {
if (!GetUtils.isEmail(value)) {
return 'Invalid Email';
}
return null;
}
String? validatePassword(String value) {
if (value.length < 6) {
return 'Password too short';
}
return null;
}
}
Use Validators in UI
Validate form inputs in UI with controller methods.
final controller = Get.put(LoginController());
TextFormField(
onChanged: (val) => controller.email.value = val,
validator: (val) => controller.validateEmail(val!),
);
TextFormField(
onChanged: (val) => controller.password.value = val,
validator: (val) => controller.validatePassword(val!),
);
9. Themes
Setup Themes
Configure light, dark, and system themes in GetMaterialApp.
GetMaterialApp(
theme: ThemeData.light(),
darkTheme: ThemeData.dark(),
themeMode: ThemeMode.system,
);
Switch Themes
Change app themes dynamically with Get.changeTheme() or
Get.changeThemeMode().
Get.changeTheme(ThemeData.dark());
Get.changeThemeMode(ThemeMode.dark);
10.Bindings
Create Binding
Define a binding class to inject dependencies for routes.
class HomeBinding extends Bindings {
@override
void dependencies() {
Get.lazyPut(() => HomeController());
}
}
Use Binding in Route
Attach bindings to routes for automatic dependency
management.
GetPage(
name: '/home',
page: () => HomePage(),
binding: HomeBinding(),
);
11.Middleware
Create Middleware
Define middleware to handle route navigation conditions.
class AuthMiddleware extends GetMiddleware {
@override
RouteSettings? redirect(String? route) {
if (!isLoggedIn) {
return RouteSettings(name: '/login');
}
return null;
}
}
Use Middleware in Route
Attach middleware to routes for access control.
GetPage(
name: '/profile',
page: () => ProfilePage(),
middlewares: [AuthMiddleware()],
);
12.Storage
Get Storage Setup
Add get_storage package for local storage capabilities.
dependencies:
get_storage: ^2.0.3
Initialize Storage
Initialize GetStorage before running the app.
void main() async {
await GetStorage.init();
runApp(MyApp());
}
Use Storage
Store and retrieve data using GetStorage
final box = GetStorage();
box.write('key', 'value');
var value = box.read('key');
13. Service
Create Service
Define a service class extending GetxService for app-wide use.
class ApiService extends GetxService {
Future init() async {
// Initialize service
return this;
}
}
Use Service
Initialize and use services with Get.putAsync().
final apiService = Get.put(ApiService());
// or with async initialization
void main() async {
await Get.putAsync(() => ApiService().init());
runApp(MyApp());
}
14.Utils
Show Custom Snackbar
Customize snackbars with colors and positions using Get.snackbar()
Get.snackbar(
'Hello',
'Snackbar with custom style',
snackPosition: SnackPosition.BOTTOM,
backgroundColor: Colors.green,
colorText: Colors.white,
);
Show Custom Dialog
Use Get.defaultDialog() to create highly customizable dialogs.
Get.defaultDialog(
title: 'Custom Dialog',
middleText: 'This is a custom dialog',
textConfirm: 'OK',
textCancel: 'Cancel',
confirmTextColor: Colors.white,
onConfirm: () => Get.back(),
);
Reactive List
Use .obs lists and Obx to reactively manage list states in UI.
var items = [].obs;
items.add('Item 1'); // Adds item reactively
Obx(() => ListView.builder(
itemCount: items.length,
itemBuilder: (context, index) {
return ListTile(title: Text(items[index]));
},
));
15. Translations
Setup Translations
Define multi-language support with the Translations class.
class MyTranslations extends Translations {
@override
Map> get keys => {
'en_US': {'hello': 'Hello'},
'es_ES': {'hello': 'Hola'},
};
}
Use Translations
Configure translation settings in GetMaterialApp
GetMaterialApp(
translations: MyTranslations(),
locale: Locale('en', 'US'),
fallbackLocale: Locale('en', 'US'),
);
Change Language
Dynamically change language using Get.updateLocale().
Get.updateLocale(Locale('es', 'ES'));
16. Workers (Listeners)
Workers
Use ever, once, debounce, and interval to listen to reactive
changes.
- ever: Called every time the variable changes.
- once: Called only the first time the variable changes.
- debounce: Called when the user stops typing for a certain amount of time.
- interval: Called at specific intervals when the variable changes.
@override
void onInit() {
ever(count, (_) => print("Count changed: $count"));
debounce(searchText, (_) => performSearch(), time: Duration(seconds: 1));
super.onInit();
}
17. Advanced Controllers
Mixin Example
Use StateMixin for handling loading, success, and error states in
controllers.
class MyController extends GetxController with StateMixin {
void fetchUser() async {
try {
change(null, status: RxStatus.loading());
User user = await ApiService.fetchUser();
change(user, status: RxStatus.success());
} catch (e) {
change(null, status: RxStatus.error(e.toString()));
}
}
}
Use Mixin in UI
Display different UI states based on controller’s status
GetX(
builder: (controller) {
if (controller.status.isLoading) {
return CircularProgressIndicator();
} else if (controller.status.isError) {
return Text('Error: ${controller.status.errorMessage}');
} else {
return Text('User: ${controller.state.name}');
}
},
);
#Tips
Shortcuts
- Get.to() instead of Navigator.push()
- Get.off() for Navigator.pushReplacement()
- Get.offAll() for Navigator.pushAndRemoveUntil()
Reactive Variables
- .obs makes a variable observable.
- Use value to get/set values: count.value = 5;
Controller Lifecycle
- onInit(), onReady(), onClose()
#Extra Tips
Use GetX for Fine-Grained Control
Rebuild specific widgets reactively with GetX.
GetX(
builder: (controller) {
return Text('Count: ${controller.count}');
},
);
Use GetBuilder for Non-Reactive Updates
Efficiently update UI without reactivity using GetBuilder.
GetBuilder(
builder: (controller) {
return Text('Count: ${controller.count}');
},
);
LazyPut with Tagging
Tag controllers for unique retrieval using Get.lazyPut() with tag.
Get.lazyPut(() => Controller(), tag: 'uniqueTag');
final controller = Get.find(tag: 'uniqueTag');