Flutter Widget Cheat Sheet: Your Ultimate Guide to Building Stunning UI
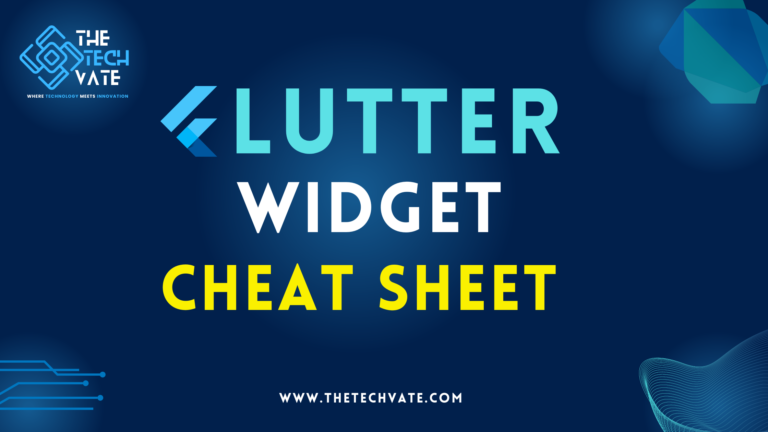
Welcome to the ultimate Flutter widget cheat sheet! In this blog post, I will share the Flutter widget cheat sheet that will help you to remember the Flutter’s basic essential widgets. It will enhance your Flutter Development Journey.
TABLE OF CONTENTS
ToggleFlutter Widget Cheat Sheet PDF
Download the PDF for Free
1. Basic Layout widget
Scaffold:
A widget that provides a framework for implementing the basic material design visual layout structure. It typically includes an app bar, a body, and other optional features like drawers and floating action buttons.
Scaffold(
appBar: AppBar(
title: Text('My App'),
),
body: Center(
child: Text('Hello, Flutter!'),
),
)
Container:
A child widget is wrapped by this widget. It features properties like alignment, padding, decoration, width, and height. It can be decorated with different shapes, borders, shadows, solid or gradient colors, and so on.
Container(
decoration: BoxDecoration(),
padding: EdgeInsets.all(8),
alignment: Alignment.center,
child: Text('Hello, Flutter!'),
)
Column:
Column(
children: [
Text('First item'),
Text('Second item'),
],
)
Row:
A widget that sets up its children widgets horizontally. Unless you wrap it with a scrollable widget, scrolling is not possible.
Row(
children: [
Text('First item'),
Text('Second item'),
],
)
Center:
It is an align widget that centers its child widget and may adjust its own size in response to the child’s dimensions
Center(
child: Text('Hello, Flutter!'),
)
Ink:
A widget that has a material ink feature. It creates an invisible ink splash effect on interaction, such as taps.
Ink(
child: InkWell(
onTap: () {
// Handle tap
},
child: Container(
width: 100,
height: 100,
color: Colors.blue,
child: Center(
child: Text('Tap me'),
),
),
),
)
Stack:
A widget that allows you to stack multiple children on top of each other. Children are positioned relative to the top-left corner of the stack by default.
Stack(
children: [
Container(
width: 200,
height: 200,
color: Colors.blue,
),
Positioned(
top: 50,
left: 50,
child: Text('Hello'),
),
Positioned(
bottom: 50,
right: 50,
child: Text('Flutter'),
),
],
)
Align:
A widget that aligns its child within itself according to a specified alignment.
Align(
alignment: Alignment.center,
child: Text('Centered Text'),
)
Padding:
A widget that adds padding around its child.
Padding(
padding: EdgeInsets.all(20.0),
child: Text('Padded Text'),
)
Transform:
Transform.rotate(
angle: math.pi / 4,
child: Text('Rotated Text'),
)
2. Widgets for responsiveness:
Expanded:
A widget that expands a child of a Row, Column, or Flex so that the child fills the available space along the main axis.
Row(
children: [
Expanded(
child: Container(color: Colors.blue),
),
Expanded(
child: Container(color: Colors.green),
),
],
)
MediaQuery:
A widget that provides information about the current app dimensions, such as screen size and orientation.
final screenWidth = MediaQuery.of(context).size.width;
final screenHeight = MediaQuery.of(context).size.height;
Flexible:
A widget that adjusts its size to fit the available space based on the flex factor.
Row(
children: [
Flexible(
flex: 2,
child: Container(color: Colors.blue),
),
Flexible(
flex: 1,
child: Container(color: Colors.green),
),
],
)
LayoutBuilder:
A widget that provides the parent widget’s constraints to its builder function, allowing you to create layouts based on the available space.
LayoutBuilder(
builder: (context, constraints) {
if (constraints.maxWidth > 600) {
return DesktopLayout();
} else {
return MobileLayout();
}
},
)
AspectRatio:
A widget that maintains a desired aspect ratio by resizing its child to fit within the available space while preserving the aspect ratio.
AspectRatio(
aspectRatio: 16 / 9,
child: Container(color: Colors.blue),
)
FractionallySizedBox:
A widget that sizes its child to a fraction of the available space in both width and height.
FractionallySizedBox(
widthFactor: 0.5,
heightFactor: 0.3,
child: Container(color: Colors.blue),
)
3. Basic Interaction Widget:
GestureDetector:
A widget that detects gestures made by the user, such as taps, drags, and swipes.
GestureDetector(
onTap: () {
// Handle tap
},
child: Container(
color: Colors.blue,
child: Text('Tap me'),
),
)
InkWell:
A widget that provides a visual ink splash effect when tapped.
InkWell(
onTap: () {
// Handle tap
},
child: Container(
color: Colors.blue,
child: Text('Tap me'),
),
)
Draggable:
A widget that allows users to drag and move its child within the parent widget.
Draggable(
child: Container(
width: 100,
height: 100,
color: Colors.blue,
child: Center(child: Text('Drag me')),
),
feedback: Container(
width: 100,
height: 100,
color: Colors.blue.withOpacity(0.5),
child: Center(child: Text('Dragging')),
),
childWhenDragging: Container(),
)
CheckBox:
A widget that represents a checkbox which users can toggle between checked and unchecked states.
Checkbox(
value: _value,
onChanged: (value) {
setState(() {
_value = value;
});
},
)
4. Basic Scrolling Widgets:
ListView:
A scrollable list of widgets arranged sequentially either vertically or horizontally.
ListView(
children: [
ListTile(title: Text('Item 1')),
ListTile(title: Text('Item 2')),
],
)
GridView:
A scrollable grid of widgets arranged in a two-dimensional layout.
GridView.count(
crossAxisCount: 2,
children: [
Text('Item 1'),
Text('Item 2'),
],
)
SingleChildScrollView:
A scrollable container that can hold only one child widget.
SingleChildScrollView(
child: Text('Scroll me!'),
)
PageView:
A scrollable list of pages, where each page occupies the full screen.
PageView(
children: [
Container(color: Colors.blue),
Container(color: Colors.green),
],
)
CustomScrollView:
A scrollable container that allows you to create custom scrolling effects by combining multiple scrollable widgets.
CustomScrollView(
slivers: [
SliverAppBar(
title: Text('App Bar'),
floating: true,
expandedHeight: 200,
flexibleSpace: FlexibleSpaceBar(
background: Image.network('https://example.com/image.jpg', fit: BoxFit.cover),
),
),
SliverList(
delegate: SliverChildListDelegate([
ListTile(title: Text('Item 1')),
ListTile(title: Text('Item 2')),
]),
),
],
)
NestedScrollView:
A scrollable container that allows you to nest scrolling widgets inside each other, such as a ListView inside a ListView.
NestedScrollView(
headerSliverBuilder: (BuildContext context, bool innerBoxIsScrolled) {
return [
SliverAppBar(
title: Text('App Bar'),
floating: true,
pinned: true,
expandedHeight: 200,
flexibleSpace: FlexibleSpaceBar(
background: Image.network('https://example.com/image.jpg', fit: BoxFit.cover),
),
),
];
},
body: ListView.builder(
itemCount: 100,
itemBuilder: (BuildContext context, int index) {
return ListTile(title: Text('Item $index'));
},
),
)
5. Text Widgets:
Text:
A widget used to display a simple text on the screen.
Text('Hello, Flutter!')
RichText:
A widget used to display text with multiple styles and formatting options.
RichText(
text: TextSpan(
text: 'Hello',
style: TextStyle(color: Colors.blue),
children: <TextSpan>[
TextSpan(text: ' Flutter', style: TextStyle(fontWeight: FontWeight.bold)),
],
),
)
SelectableText:
A widget used to display text that can be selected by the user.
SelectableText('Selectable Text')
6. Image Widget:
Image.asset:
A widget that displays an image included in your asset bundle.
Image.asset('assets/image.png')
Image.network:
A widget that displays an image obtained from a URL.
Image.network("Your Image URL");
FadeInImage:
A widget used to display a placeholder image while loading the final image from the network.
FadeInImage.assetNetwork(
placeholder: 'assets/placeholder.png',
image: 'https://example.com/image.jpg',
)
CircleAvatar:
A widget used to display a circular avatar image, commonly used for user profile pictures.
CircleAvatar(
backgroundImage: AssetImage('assets/avatar.png'),
radius: 50,
)
7. Input and Controls:
TextField:
A widget used to allow users to input text.
TextField(
decoration: InputDecoration(
labelText: 'Enter your name',
),
)
Slider:
A widget used to allow users to select a value from a range by sliding a thumb along a track.
Slider(
value: _sliderValue,
onChanged: (value) {
setState(() {
_sliderValue = value;
});
},
)
Switch:
A widget used to allow users to toggle between two states, typically on/off.
bool _value = false;
Switch(
value: _value,
onChanged: (value) {
setState(() {
_value = value;
});
},
)
Elevated Button:
A material design elevated button, when pressed, it elevates and displays ink reactions.
ElevatedButton(
onPressed: () {
// Handle button press
},
child: Text('Press Me'),
)
Text Button:
A material design text button, typically used for less prominent actions.
TextButton(
onPressed: () {
// Handle button press
},
child: Text('Press Me'),
)
Outlined Button:
A circular material design floating action button, typically used for primary actions in an app.
OutlineButton(
onPressed: () {
// Handle button press
},
child: Text('Press Me'),
)
FloatingAction Button:
A circular material design floating action button, typically used for primary actions in an app.
FloatingActionButton(
onPressed: () {
// Handle button press
},
child: Icon(Icons.add),
)
Icon Button:
A button widget that displays an icon, typically used for actions in an app bar or toolbar.
IconButton(
icon: Icon(Icons.star),
onPressed: () {
// Handle button press
},
)
Conclusion:
Scaffold
, Container
, Column
, and Row
to more advanced widgets for responsiveness like Expanded
, Flexible
, andMediaQuery
, we explored how to create visually appealing and adaptable UIs. TextField
, Checkbox
, andSlider
, as well as widgets for creating interactive elements like GestureDetector
, InkWell
, andDraggable
.ListView
, GridView
, SingleChildScrollView
, PageView
, CustomScrollView
, and NestedScrollView
. With these tools at your disposal, you can efficiently develop robust and engaging Flutter applications. If you have any further questions or suggestions for additional topics, feel free to reach out!FAQ
1. What is the difference between GestureDetector and InkWell?
- Both widgets detect user gestures like taps, but
InkWell
provides a visual ink splash effect when tapped, whileGestureDetector
does not.
2. How can I create responsive layouts in Flutter?
- Flutter provides several widgets like
Expanded
,Flexible
,MediaQuery
,LayoutBuilder
,AspectRatio
, andFractionallySizedBox
to create responsive layouts that adapt to different screen sizes and orientations.
3.What is the purpose of the Transform widget?
- The
Transform
widget allows you to apply transformations like rotation, scaling, or translation to its child widget, enabling you to create various visual effects.
4. How do I handle user input in Flutter?
- User input can be handled using widgets like
TextField
for text input,Checkbox
for toggling between checked and unchecked states,Slider
for selecting values from a range, andSwitch
for toggling between two states.
5. What widgets can I use for scrolling content in Flutter?
- For scrolling content, you can use widgets like
ListView
for sequentially arranged lists,GridView
for grid layouts,SingleChildScrollView
for a single child that needs scrolling,PageView
for a list of full-screen pages,CustomScrollView
for custom scrolling effects, andNestedScrollView
for nesting scrolling widgets.